何度も過去、以下を書いたが、Wicket ModalWindow 内の height の調整が抜けていた。。
Wicket full size ModalWindow - Oboe吹きプログラマの黙示録
Wicket ModalWindow full screen - Oboe吹きプログラマの黙示録
heightの調整、先に答えになる JS (jQuery)ソース全体を書くと、、、
var _adjust_full_nocaption = function(){
$(".wicket-modal").attr("style", "top:0;left:0;width:100%;position:fixed;visibility:visible;"),
$(".w_content_container").attr("style", "overflow:auto;height:" + window.innerHeight + "px;");
$(".w_content_container div div").attr("style", "height:" + window.innerHeight + "px;");
$("div.w_caption").attr("style", "display:none;"),
$("div.w_top_1").attr("style", "display:none;"),
$("div.w_bottom_1").attr("style", "display:none;"),
$("div.w_right_1").attr("style", "margin:0;"),
$("div.w_content_1").attr("style", "margin:0;");
}
var _adjust_ful_captionON = function(){
$(".wicket-modal").attr("style", "top:0;left:0;width:100%;position:fixed;visibility:visible;"),
$(".w_content_container").attr("style", "overflow:auto;height:"+ (window.innerHeight - 10) + "px;");
$(".w_content_container div div").attr("style", "height:" + (window.innerHeight - 10) + "px;");
}
var adjustFull = function(msec){
setTimeout("_adjust_full_nocaption()", msec);
$(window).off('resize');
$(window).on('resize', function(){
_adjust_full_nocaption();
});
};
var adjustFullHandle = function(msec){
setTimeout("_adjust_ful_captionON()", msec);
$(window).off('resize');
$(window).on('resize', function(){
_adjust_ful_captionON();
});
};
ModlalWindow を開いたら実行する関数
adjustFull ( 10 ) ; ・・・・10 msec 後に描画サイズ調整
または、
adjustFullHandle ( 10 ) ; ・・・・10 msec 後に描画サイズ調整
_adjust_full_nocaption 関数で、
$(".w_content_container div div").attr("style", "height:" + window.innerHeight + "px;");
_adjust_ful_captionON 関数で、
$(".w_content_container div div").attr("style", "height:" + (window.innerHeight - 10) + "px;");
が足りなかった。
これは、ModalWindow が以下のHTML要素で描画されているからだ、
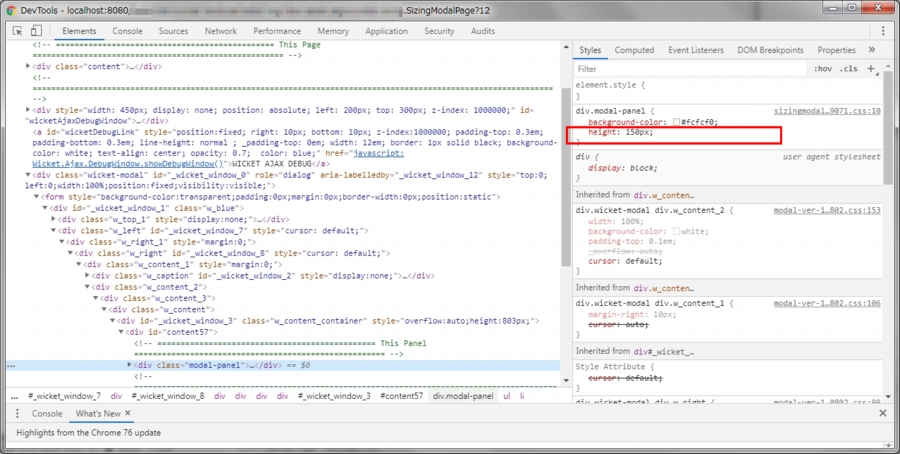
class="wicket-modal" の <div> の中に、
<div class="w_top_1" >
<div class="w_left" >
が存在して、
<div class="w_left" > の中に、
<div class="w_right_1" >
<div class="w_right" >
<div class="w_content_2" >
<div class="w_content_3" >
<div class="w_content" >
<div class="w_content_container" >
があってようやくこの中に、Panel 生成の div 1個で括って、
Panel として用意する div が生成される。
<wicket:panel>
<div class="modal-panel">
・・・・
</div>
</wicket:panel>
この <div class="modal-panel" > が、上の深い div 階層の中に入る。
Wicket ModalWindowをページいっぱいFull に表示させることで戻りを想定するページ遷移の代わりにする。
→ セッション保持させるものを少なくする設計ができる。
・・・もはや、ModalWindow という名がふさわしくなくなるけど、魅力的な方法だ。